Vercel offers a range of powerful storage solutions designed specifically for serverless web applications. These storage products enable developers to efficiently manage and store their data, ensuring reliable and seamless performance.
One of the key offerings is Vercel KV, which provides a serverless key-value storage solution. It allows developers to store and retrieve structured data with ease. Additionally, Vercel Postgres offers a serverless SQL database option, enabling developers to store and query dynamic content for their applications.
For file storage needs, Vercel Blob is an external file storage solution. It provides a reliable and scalable option for storing files, making it easier to manage content such as images or documents.
To further enhance performance and customization, Vercel Edge Config allows developers to define edge middleware using JavaScript. This allows for ultra-low latency responses and customized caching strategies for the data stored in Vercel's storage offerings.
With these serverless storage solutions, developers have the flexibility to choose the correct storage solution for their specific use case, whether it's key-value storage, dynamic content storage, or file storage. Vercel empowers developers to build the fastest frontends by offering reliable and intuitive storage options for their serverless web applications.
How to set up & use Vercel KV (Redis database)
The developer experience with Vercel KV is seamless and efficient, thanks to its integration with Upstash, a powerful key-value storage provider. Leveraging Upstash, Vercel KV delivers durable storage and persistence on disk by default, ensuring that developers can rely on the stability and longevity of their data.
The key benefit of Vercel KV is its ability to persist state without data loss. This is especially crucial for applications that require caching and persistent data storage, as it ensures that no valuable information is lost even during server reboots or application updates.
Vercel KV's user-friendly interface and intuitive APIs make it easy for developers to interact with and manage their data. Its promise-based API simplifies the process of storing and retrieving structured data, allowing you to focus on building your applications rather than worrying about the underlying infrastructure.
In real-world scenarios, Vercel KV can greatly enhance application development. Personally I would for exampleuse it to store user session data, resulting in faster and more efficient session management. Besides that, it can be integrated into search functionalities, enabling you to quickly search through large volumes of data with minimal network roundtrips. The durability and persistence of Vercel KV make it a reliable option for storing critical information, such as user preferences or application settings.
Based on my limited testing so far, Vercel KV provides developers with a seamless experience, combining the reliability of durable key-value storage with the convenience of Upstash's disk persistence. You can confidently store and manage your data without the risk of data loss, ultimately speeding up your application development process.
Integrating Vercel KV into your Next.js 13 project
We begin by setting up an empty Next.js project.
npx create-next-app@latest vercel-kv-demo
# Answer the following prompts as you like:
# Would you like to use TypeScript? … No / Yes
# Would you like to use ESLint? … No / Yes
# Would you like to use Tailwind CSS? … No / Yes
# Would you like to use `src/` directory? … No / Yes
# Would you like to use App Router? (recommended) … No / Yes
# Would you like to customize the default import alias (@/*)? … No / Yes
Set it up to your liking. I typically choose for Tailwind, TypeScript and leave the rest default.
Once you have set up your base project, lets add this project to Vercel. Before we can do that, let's add the project to our Github. Once you've added the code to your Github repository, or made a fork of my Vercel KV demo repository, we can import it into our Vercel account. You can import the project by going to vercel.com/new
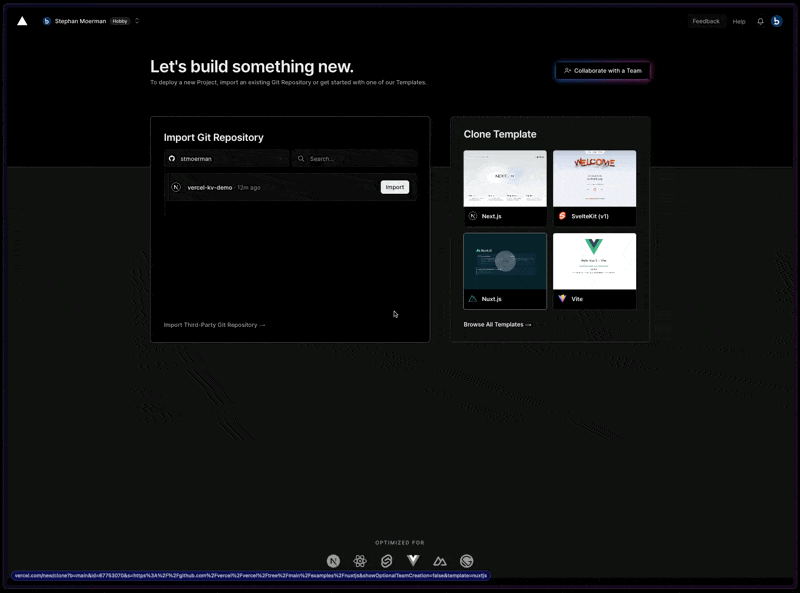
Now that we have our Vercel project initialized, we can add the Vercel KV package to our project to create a store.
Vercel KV works with any frontend framework or as a standalone Redis database. First, lets install the package:
npm i @vercel/kv
# yarn add @vercel/kv
# pnpm i @vercel/kv
Next up we need to create a new database inside our project. Let's head over to our Vercel project and create a new KV storage. During this process you can select a region and select the environments you want to use this database in. For this demo we will use the default and leave Production, Preveiw and Development checked.
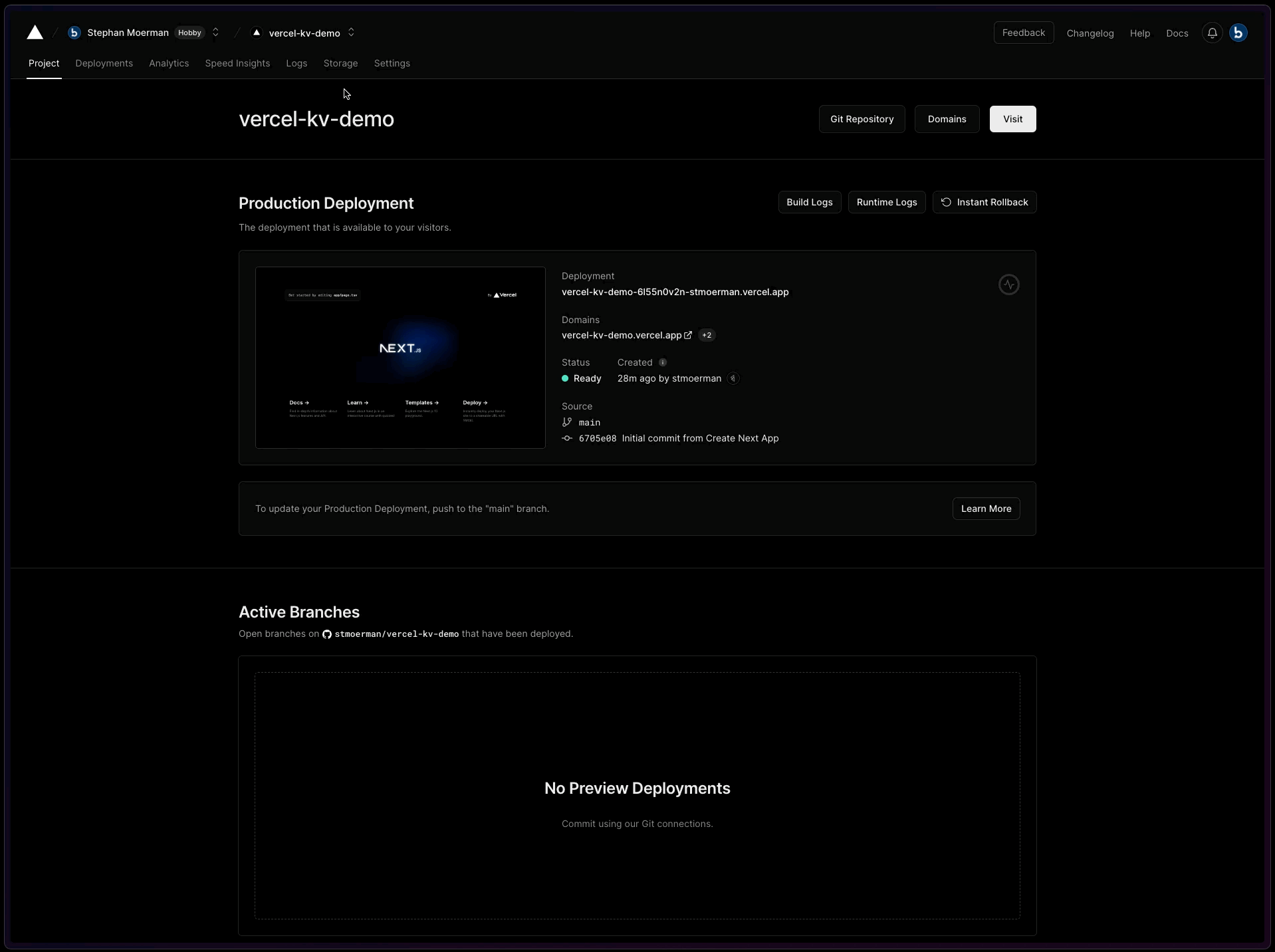
After we have set up our KV storage, Vercel automatically links it to your project. Now we can check if our environment variables were set up correctly. You can check your environment variables by clicking the Settings tab in your Vercel project. Here you can see the environment variables that were set up for you. You can also add new environment variables here. You should see the following KV environment variables:
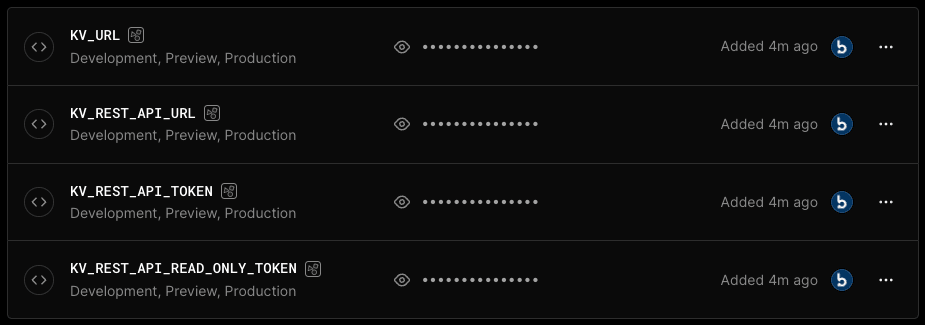
Next lets add some data into our database by populating the store using the CLI interface. You can do this by running the following command in your CLI interface in the dashboard:
hset user:me email [email protected] id 123
To access the CLI in your Vercel KV project, click the Storage tab, open up the Redis database that we created earlier and down below you'll find the CLI. This is where you can paste the command.
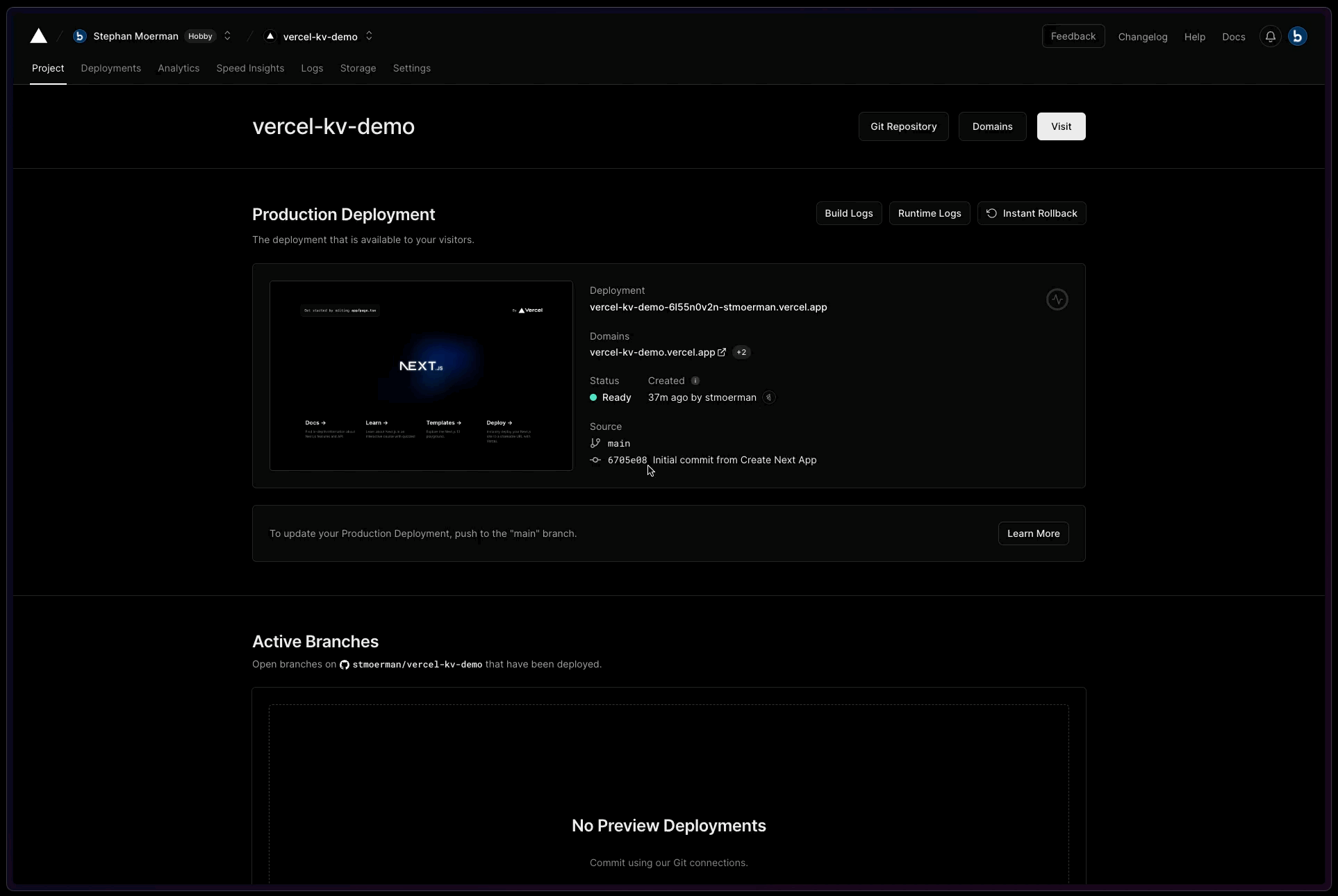
You can check your storage hash with the following command to confirm you've succesfully populated your database.
hgetall user:me
You'll get the following response:
email, [email protected], id, 123
So now we know we have some data in our database. Next we need to add the environment variables for our project locally. You can do this by using the Vercel CLI to pull the environment variables automatically:
vercel env pull .env.development.local
But if you prefer to do so, you can also copy them over manually by visiting the Storage tab, opening your KV store and clickign on .env.local
under the Quickstart. You'll find all your environment variables here and you can simply copy them into your clipbloard.
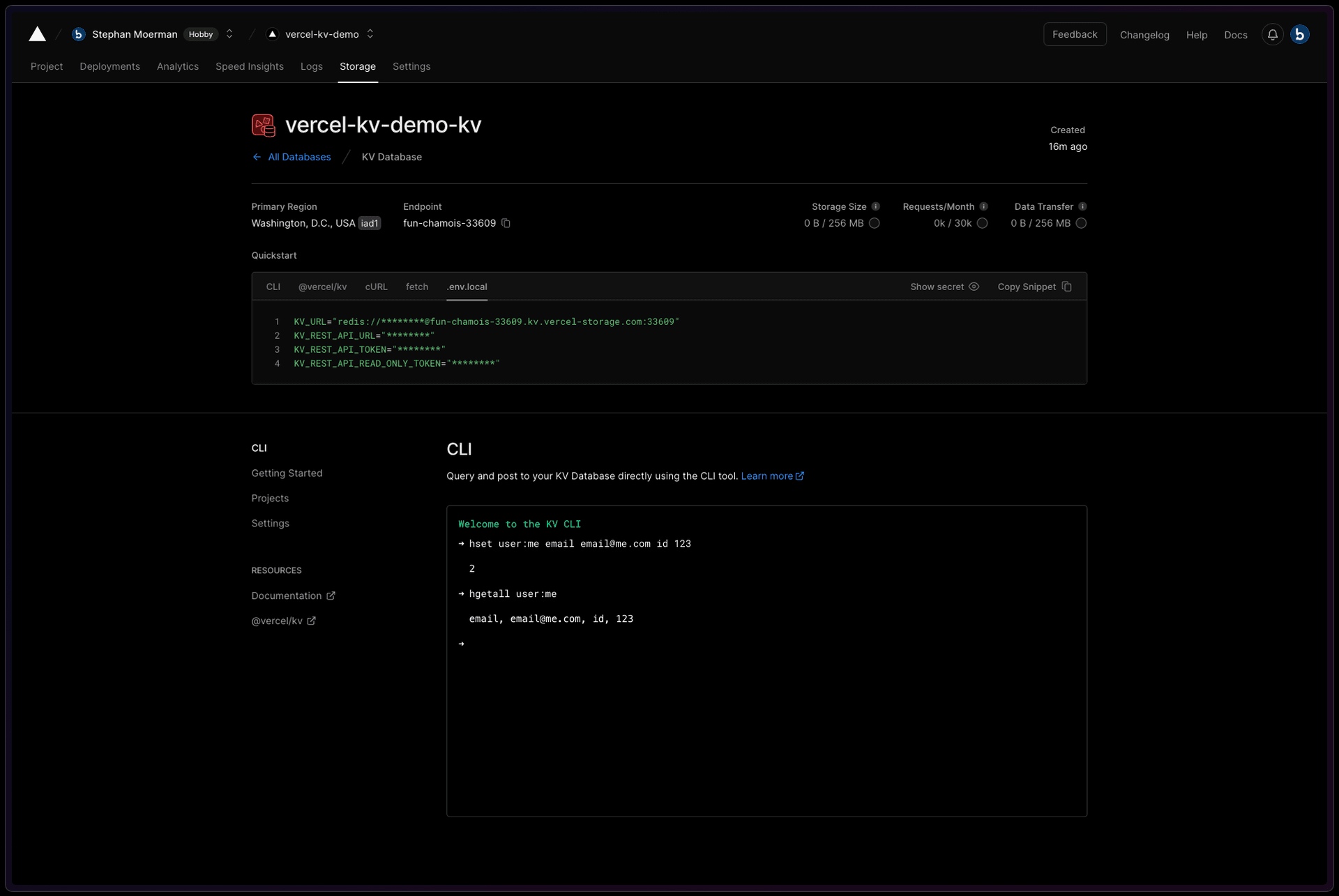
Lets create a simple API route to query our KV store. As we can now retrieve data, lets add an API route inside our app folder.
import { kv } from "@vercel/kv";
import { NextResponse } from "next/server";
export async function GET() {
const user = await kv.hgetall("user:me");
return NextResponse.json(user);
}
If we now open up a browser and visit localhost:3000/api/user we will see that the data that we populated gets returned.
{
"email": "[email protected]",
"id": 123
}
So there you go. This is a super simple example of how you can use the new Vercel KV! In a project you would of course create more advanced integrations, but this is a great way to get started. You can also integrate Vercel KV into an existing project.
Of course a key-value store is not suitable for every usecase and sometimes you might want to use something like Postgres for your application. Likely Vercel has the Postgres integration as well, so lets take a look at that next!
Vercel Postgres: how to setup & use serverless PostgreSQL database
Are you a developer looking for a seamless and efficient experience when it comes to server-side data management? Look no further than Vercel Postgres. With its array of key features and benefits, Vercel Postgres allows you to enhance your productivity and build powerful applications effortlessly.
One of the standout features of Vercel Postgres is its seamless integration with popular frameworks like Next.js App Router, Server components, Nuxt, and SvelteKit. Connecting your Next.js, Nuxt, or SvelteKit applications to Vercel Postgres has never been easier. By leveraging the robust capabilities of Postgres for data management, you can take your application to new heights.
Say goodbye to excessive client-side JavaScript with Vercel Postgres. By enabling powerful server-side data mutations, this tool drastically reduces the need for extensive client-side scripting. You can now perform complex data manipulations and transformations directly on the server, resulting in cleaner and more efficient code. This not only enhances overall performance but also improves the user experience of your application.
But that's not all. Vercel Postgres goes above and beyond to provide a seamless developer experience. Its user-friendly interface and intuitive APIs make interaction and data management a breeze. You can now focus on building your applications without worrying about the complexities of database management. Vercel Postgres takes care of it all.
Vercel Postgres is the ultimate solution for developers seeking efficient server-side data management, especially if you've used PostgreSQL before. With its seamless integration, powerful data mutations, and developer-friendly design, you can build high-performance applications with ease and efficiency. Experience the difference with Vercel Postgres today.
Features | Benefits |
---|---|
Postgres integration | Seamlessly fetch data from your Postgres database |
SQL mutations | Perform powerful server-side data manipulations |
ORMs support | Easily import and write SQL strings with upcoming support for ORMs |
Server-side rendering | Render dynamic content on the server using Postgres data |
Database scalability | Scale your database as your application grows |
Integrating Vercel Postgres into your Next.js 13 project
Lets kick-off a new Next.js 13 app router project and see how easy it is to get started with Vercel Postgres. In this example we'll create a new Next.js project, but you can of course also use this for an existing project.
We begin as we always do, by setting up an empty Next.js project.
npx create-next-app@latest vercel-postgres-demo
# Answer the following prompts as you like:
# Would you like to use TypeScript? … No / Yes
# Would you like to use ESLint? … No / Yes
# Would you like to use Tailwind CSS? … No / Yes
# Would you like to use `src/` directory? … No / Yes
# Would you like to use App Router? (recommended) … No / Yes
# Would you like to customize the default import alias (@/*)? … No / Yes
You can now repeat the previous steps, and add the code to a Github repository and import it into Vercel.
Once you've done that, you are ready to install the @vercel/postgres
package.
npm i @vercel/postgres
# yarn add @vercel/postgres
# pnpm i @vercel/postgres
We can now navigate to the project we just created on Vercel, and click on the Storage tab to start setting up Vercel Postgres.
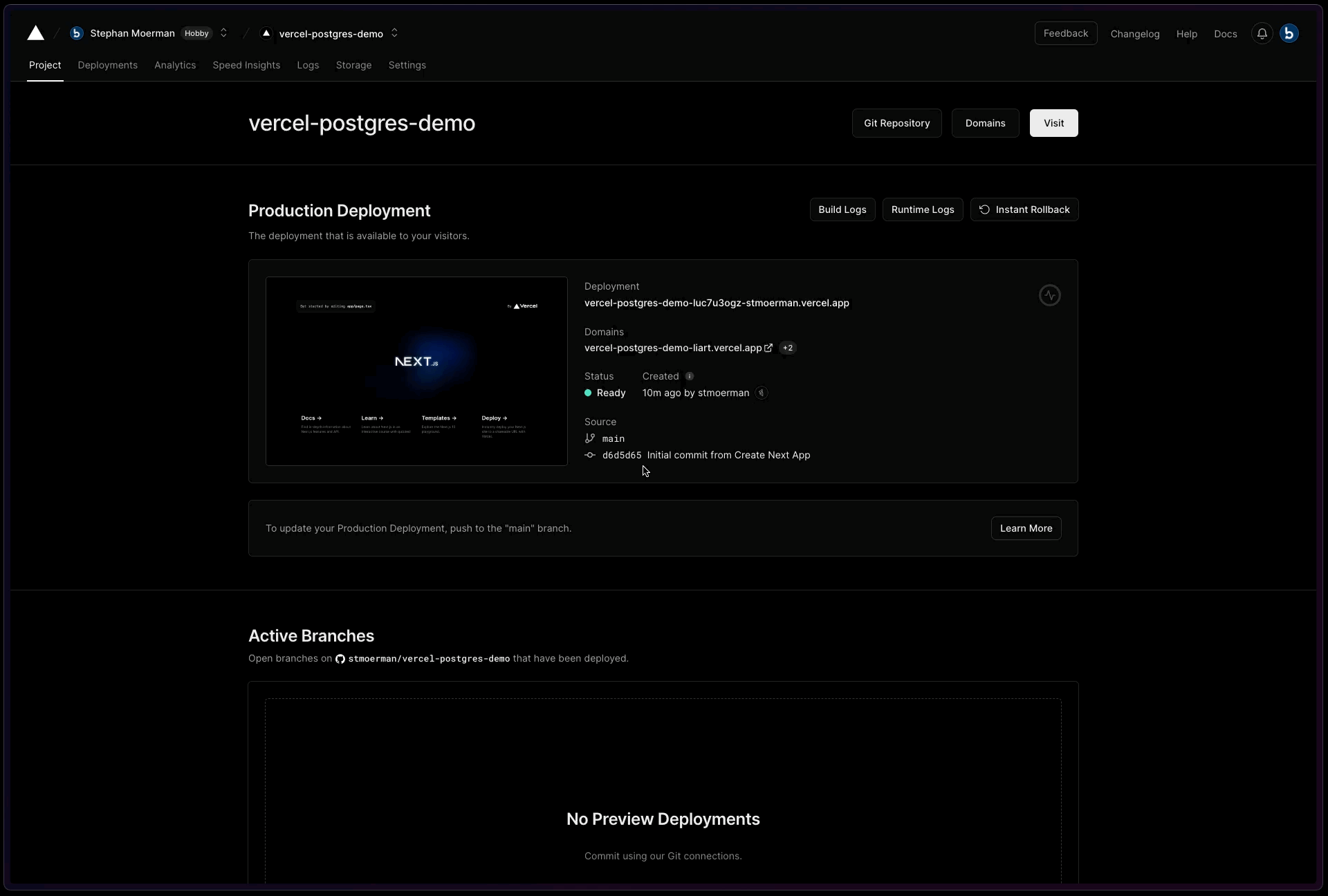
As with Vercel KV, you can now check your environment variables and you should see the following added:
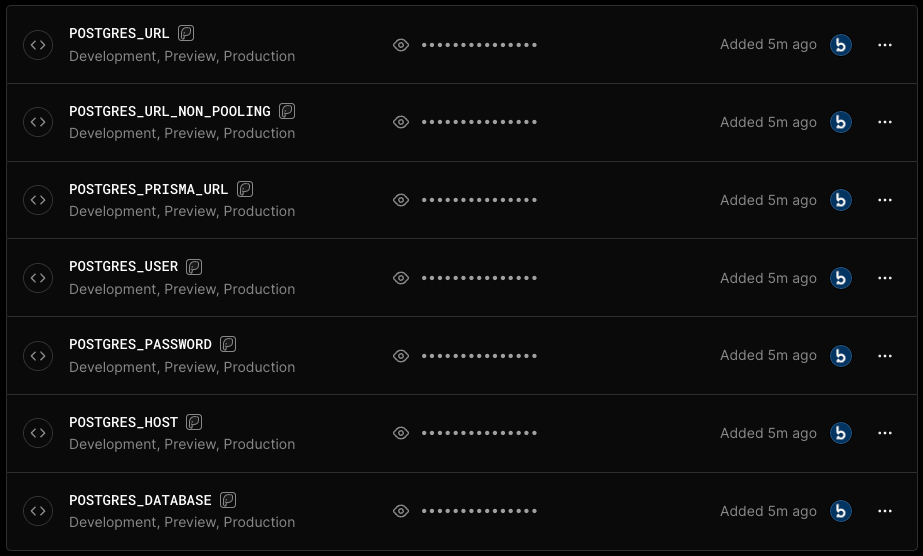
Lets copy the environment variables into our Next.js project. You can do this by using the Vercel CLI to pull the environment variables automatically:
vercel env pull .env.development.local
Or manually copying them from within the Storage tab into your .env.development.local
file with the following values:
POSTGRES_URL="*******************"
POSTGRES_PRISMA_URL="*******************"
POSTGRES_URL_NON_POOLING="*******************"
POSTGRES_USER="*******************"
POSTGRES_HOST="*******************"
POSTGRES_PASSWORD="*******************"
POSTGRES_DATABASE="*******************"
We are ready to test our integration so let's populate the database with some data. To do so, we're going to create an API route inside our app
folder.
import { sql } from "@vercel/postgres";
import { NextResponse } from "next/server";
export async function GET(request: Request) {
try {
const result =
await sql`CREATE TABLE Demo ( Name varchar(255), Owner varchar(255) );`;
return NextResponse.json({ result }, { status: 200 });
} catch (error) {
return NextResponse.json({ error }, { status: 500 });
}
}
You can now run next dev
or npm run dev
to start your app locally.
We can now create the Demo
table by visiting our API route: localhost:3000/api/create-table
You should see something like this in your browser as a response on the API page:
{
"result": {
"command": "CREATE",
"fields": [],
"rowAsArray": false,
"rowCount": null,
"rows": [],
"viaNeonFetch": true
}
}
So thats a super simple example on how to get started with using Vercel Postgres. You can now use this to create a table and start populating it with data. You can also use this to query data from your database.
Next up, lets take a look at Vercel blob and when you should consider using Vercel blob.
Vercel blob: an intuitive solution for reliable file storage and delivery
Are you in search of a hassle-free solution to host large static files on the same platform? Look no further than Vercel Blob! It offers a surefire way to meet all your file uploading and sharing needs. With Vercel Blob, you can say goodbye to the time-consuming setup and management of external storage buckets like AWS S3, DigitalOcean Space, and more. Find convenience and efficiency in one place!
With Vercel Blob, you can enjoy the following benefits:
- Seamless integration: Vercel Blob smoothly integrates into your workflow, making it easy to upload and manage files.
- Versatility: Whether you need to store avatars, screenshots, cover images, videos, or even large files like audios, Vercel Blob has got you covered.
- Global network advantage: Take advantage of Vercel Blob's global network to ensure fast and efficient delivery of your files.
- Cost-effective solution: Instead of relying on external file storage services like Amazon S3, you can leverage Vercel Blob to access and manage your files effortlessly.
Getting started with Vercel blob
To start using Vercel Blob, simply create and manage your Blob stores from your account dashboard. You can effortlessly connect them to as many projects as you want, ensuring flexibility and scalability.
Server and client uploads
Uploading files to Vercel Blob is a breeze, and you have two options to choose from:
- Server uploads: The most common method, where the file is first sent to your server and then to Vercel Blob. While it's simple to implement, please be aware that there is a limit on the file size of 4.5 MB for Vercel-hosted websites.
- Client uploads: This advanced solution allows you to securely send files directly from the client (e.g., a browser) to Vercel Blob. It offers more flexibility, enabling you to upload files up to 500 MB.
Note: You can also upload files larger than 4.5 MB directly from a script or server code, as long as they are not received from a Vercel-hosted website.
There's more to come soon though, as Vercel is working on some exciting features, such as Private blob capabilities. Soon you'll be able to make blobs private, ensuring an added layer of security for your files.
For specific queries regarding integration into your workflow, it is important to understand that there are some workflow considerations:
- Each Vercel account can have multiple Blob stores.
- Multiple Vercel Blob stores can be used within one Vercel project.
- Vercel Blob stores can be accessed by multiple Vercel projects.
- Vercel Blob URLs are publicly accessible, generated with an unguessable random ID, and immutable.
- Adding or removing content from a Blob store requires a valid token.
I hope you enjoy using Vercel Blob as much as I do. Happy uploading!
Limitations of Vercel blob storage
Vercel Blob Storage, although a powerful and reliable solution for static file storage, has some limitations and constraints that users should be aware of. Firstly, it is important to note that Vercel Blob is currently only available in Beta on Hobby and Pro plans. While this allows early access to the platform and the opportunity to provide valuable feedback, it may also mean that certain features and capabilities are still being developed and refined.
Additionally, Vercel Blob has certain constraints on file sizes and storage limits. Depending on the account plan type, there may be restrictions on the maximum file size that can be uploaded and the total amount of storage available. Users should carefully review these constraints to ensure that Vercel Blob meets their specific requirements.
When comparing Vercel Blob with other storage solutions, such as Theo's UploadThing, it's important to consider the specific needs and preferences of your project. While both solutions offer file storage capabilities, they may differ in terms of features, pricing, and integration options. Users should evaluate their own requirements and consider factors such as availability, scalability, and integration with their existing tech stack before making a decision.
Pricing and cost considerations
When considering Vercel's storage products, it's important to take into account the pricing and cost considerations. Vercel offers different pricing tiers to cater to various needs.
The pricing tiers include Basic, Pro, and Enterprise, each offering different levels of features and limits. Basic operations, such as reads, writes, deletes, and request handling, are included in all tiers. Advanced operations, like streaming uploads and ranged reads, are available in the Pro and Enterprise tiers.
Storage limits also vary across the tiers, ranging from 5GB in the Basic tier to 1TB in the Enterprise tier. Data transfer limits are also different for each tier, ensuring scalability and flexibility.
It's worth noting that blob downloads are not counted towards basic operations when served from the edge cache, providing an additional benefit in terms of cost savings.
By understanding the different pricing tiers and their respective included operations, storage, and data transfer limits, users can select the most suitable and cost-effective option for their specific requirements.
Convenience and benefits of Vercel's storage products
Vercel's storage products offer a convenient and efficient solution for developers looking to incorporate storage capabilities into their web applications. With a range of options available, including Vercel KV, Vercel Postgres, Vercel Blob, and Vercel Edge Config, developers have access to a comprehensive suite of storage solutions.
One of the key benefits of Vercel's storage products is their seamless integration with Vercel's platform for frontend developers. This integration simplifies the deployment and management process, allowing developers to focus on their code rather than infrastructure.
Vercel KV provides durable Redis servers, offering a reliable and high-performance key-value storage solution. Vercel Postgres enables developers to utilize serverless SQL servers, providing dynamic content and efficient database management. Vercel Blob is ideal for large file storage, ensuring that developers have the necessary resources for their projects. Additionally, Vercel Edge Config offers global, low-latency data stores, optimizing performance and enhancing user experience.
By utilizing Vercel's storage products, developers benefit from reduced complexity, improved scalability, and enhanced reliability. The intuitive and promise-based API allows for seamless interaction with the different storage solutions, ensuring that developers can quickly and easily implement the correct storage solution for their specific needs.
Overall, Vercel's storage products provide a convenient and efficient way to incorporate storage capabilities into web applications, empowering developers to build the fastest and most reliable frontends possible.
Benefits | Convenience | Ease of Use |
---|---|---|
Simplify frontend storage needs | Seamless integration with existing code bases | User-friendly experience |
Reduce frequent database queries | Suitable for various use cases | Intuitive and accessible |
Improve development workflow | Easy file storage at the edge | Efficient and reliable |
Enhance caching capabilities | Durable Redis database | Scalable and performant |
Why now? Server-first and edge-first frameworks
Server-first and edge-first frameworks have become increasingly important in the development world, and their relevance is closely tied to the use of Vercel's storage products. These frameworks prioritize server-side rendering and edge computing, enabling developers to optimize performance and deliver data more efficiently.
Server-first frameworks, such as Next.js, allow developers to render web pages on the server before sending them to the client's browser. This approach dramatically improves initial page load times and provides better search engine optimization. By leveraging Vercel KV, developers can easily store and retrieve frequently accessed data, ensuring quick access and reducing database roundtrips.
Edge-first frameworks, such as Vercel's Edge, enable developers to run their code on the edge network, closer to the user. This architecture significantly reduces latency and improves responsiveness. With the use of Vercel Blob, developers can efficiently store and retrieve large files, such as images or videos, enabling low-latency delivery to users worldwide.
By leveraging Vercel KV, Vercel Postgres, and Vercel Blob as integral components of server-first and edge-first architectures, developers can optimize performance and deliver data more efficiently. These storage products seamlessly integrate with Vercel's platform, reducing complexity and improving scalability. With a reliable and highly performant storage solution, developers can focus on crafting the fastest frontends and delivering an exceptional user experience.
Seamless Redis integration: the power of Vercel KV
Vercel KV stands out as a durable Redis database that offers an array of features and benefits. As a serverless and Redis-compatible solution, it provides developers with an easy-to-use and highly durable database, allowing them to create Redis-compatible databases that can be written to and read from Vercel's Edge Network.
One of the key advantages of Vercel KV is its durability. It leverages the Vercel platform's robust infrastructure, ensuring that data is persisted in memory and on disk by default. This means that even in the event of server crashes, data remains safe and accessible.
Working in conjunction with Upstash, Vercel KV enables developers to seamlessly leverage Redis functionality within the Vercel ecosystem. The partnership ensures that developers can take full advantage of the power and flexibility of Redis databases while benefiting from Vercel's simplified deployment and scaling capabilities.
Furthermore, Vercel KV is designed to be user-friendly and straightforward to implement. With its intuitive and promise-based API, developers can easily store and retrieve data, making it an ideal choice for developers of all skill levels.
In summary, Vercel KV is a durable Redis database that is serverless, Redis-compatible, easy to use, and highly durable. It empowers developers to create and manage Redis-compatible databases within the Vercel ecosystem, providing a reliable and efficient solution for their data storage needs.
Vercel Postgres: next-gen data handling for superior performance
Vercel Postgres is a serverless SQL database designed specifically for the frontend cloud. It offers developers a fully managed, scalable, and fault-tolerant solution for handling complex relational data.
One of the key benefits of Vercel Postgres is its compatibility with popular frameworks like Next.js, Nuxt, and SvelteKit. This allows developers to seamlessly integrate the database with their frontend applications, making it easy to fetch data from the server and render dynamic content.
With server-first data mutations, Vercel Postgres simplifies the process of updating and modifying data. This reduces the reliance on client-side JavaScript and improves performance.
Vercel Postgres also provides a simple API that makes working with complex data straightforward. Developers can easily store and retrieve data, without worrying about managing the underlying infrastructure.
With that being said. I think Vercel Postgres perfect solution for developers working in the frontend cloud who need a powerful and scalable serverless SQL database. Its compatibility with popular frameworks and its simplicity make handling complex data a breeze.
You can manage complex data easily with Vercel Postgres:
-
Server side rendering with Vercel Postgres: You can fetch data from your Postgres database to render dynamic content on the server, reducing the need for client-side JavaScript.
-
Scalability and fault tolerance with Vercel Postgres: It's fully managed, highly scalable, and fault-tolerant, ensuring your database can handle increased traffic and maintain uptime.
-
Migrating to Vercel Postgres from other SQL databases: Vercel provides easy migration tools and documentation to help you seamlessly transition your data from other SQL databases.
-
Leveraging Vercel Postgres for server first data mutations: Vercel Postgres allows for powerful server-first data mutations, enabling you to perform complex operations on the server side.
-
Integrating Vercel Postgres with Next.js, Nuxt, and SvelteKit: Vercel Postgres integrates seamlessly with popular frameworks like Next.js, Nuxt, and SvelteKit, providing a smooth development experience.
Conclusion
In conclusion, Vercel Storage offers a reliable and efficient solution for all your file storage needs. Whether you choose KV, Postgres, or Blob, Vercel provides convenience and benefits that can revolutionize your file storage experience.
One interesting statistic to note is that Vercel Blob enables global distribution of large files, allowing for faster and more efficient access to your data.
With Vercel Storage, you can trust that your files are securely stored and easily accessible whenever you need them.
Do you want any help with integrating Vercel Storage into your workflow/application? Let's connect!